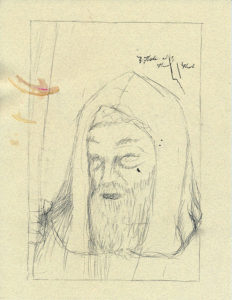
This approach more easily accommodates future requirements changes that would otherwise require a complete restructuring of business-domain classes in the inheritance model. The one and only resource you'll ever need to learn APIs: Want to kick start your web development in C#? To compose a class from existing classes, an object of each class should be declared as the member of the new class. If the features of one object need to be a part of another object, the relationship calls for composition. If the features of one object need to be a part of another object, the relationship calls for composition. Even when we can use inheritance, it doesnt mean we should use inheritance. If X is Y, class X can inherit from class Y. In contrast, inheritance does not require all of the base class's methods to be re-implemented within the derived class. This hierarchy is fragile, and the implementation of derived classes can break or be forced to change with any change at the top of the hierarchy. By establishing a relationship between new and existing classes, a new class can inherit or embed the code from one or more existing classes. By implementing inheritance, member functions from one class become properties of another class without coding them explicitly within the class. However, its not viable to re-write the complete code on introducing a change once its deployed. Introduce an abstract class named VisibilityDelegate, with the subclasses NotVisible and Visible, which provides a means of drawing an object: Introduce an abstract class named UpdateDelegate, with the subclasses NotMovable and Movable, which provides a means of moving an object: Introduce an abstract class named CollisionDelegate, with the subclasses NotSolid and Solid, which provides a means of colliding with an object: Finally, introduce a class named Object with members to control its visibility (using a VisibilityDelegate), movability (using an UpdateDelegate), and solidity (using a CollisionDelegate). class KickingMonster() : Monster { private readonly ICanKick kick; public KickingMonsterICanKick damage) { this.kick= kick; } }. So i think this i much more flexible and readable. You might have heard the saying Favor object composition over class inheritance Some programming languages like GO and rust are designed to facilitate composition over inheritance. This is where you use composition. Using ReSharper for clean and consistent C# code, https://ericlippert.com/?s=Wizards+and+warriors&submit=Search, Coding with Scott (programming tutorials), Naucz si C#, tworzc prost gr RPG (Polish version), Life as a programmer outside Silicon Valley, Add a new Spitting value to the AttackType enum in Monster.cs, Add a new CanSpit property to Monster.cs, Add a new SpitDamage property to Monster.cs. Alternative implementation of system behaviors is accomplished by providing another class that implements the desired behavior interface. With this Monster class, when we create a new Monster object, we compose its Attack options by calling the AddAttackType() function with the AttackType, and the amount of damage the monster does with this attack. With inheritance, you can create classes that derive their attributes from existing classes so while using inheritance to create a class, you can expand on an existing class. Composition also provides a more stable business domain in the long term as it is less prone to the quirks of the family members. This was so funny and clever, had to pause for a moment when I noticed it. Thus, along with our previous criteria, we need to consider the Liskov Substitution Principle, which says, the base class objects should be replaceable with the derived class objects and this replacement should not break the application.. Since you can only extend one class in Java, but if you need multiple features, such as reading and writing character data into a file, you need Reader and Writer functionality. Nevertheless, it is often preferable to use composition over inheritance. To put it simply, when a derived class inherits from a base class, it acquires properties and behaviors of the base class. Weve talked about both implementations, what are the differences, and is composition better than inheritance. It does not inherit from Person but instead gets the Person object passed to it, which is why it has a Person. With composition, we can choose the functionalities we want to implement in the composite class based on the components unlike inheritance, where the derived class automatically has access to all the base class methods. Additionally, composition provides a better way to use an object without compromising the internal details of the object, that is where composition is useful. While both inheritance and composition promote code reusability in object oriented system by establishing relationships between classes and they provide equivalent functionality in many ways, they use different approaches. The Employee also shadows the title property from Person, meaning Employee. To get the higher design flexibility, the design principle says that composition should be favored over inheritance. I was having a hard time grasping composition and finding a practical example, this one is fantastic to visualize better what composition is and the benefit. This is the best post Ive read on Composition over inheritance. In general, it is preferable to use composition as it provides a way to use an object without compromising the internal details of the object that is where composition is useful. A HighBite (by a dinosaur) would check its hit against the players helmet stats. This test alone might not be helpful every time. This class has methods which delegate to its members, e.g. Many a times, you might want to use an object as a field within another class. In inheritance, you specialize a class to create an is-a relationship between the classes which results in a strong coupling between the base and derived classes. These component objects should not be exposed directly as they exist only within the composite context. Class inheritance also makes it easier to modify the implementation being reused. In the above example, The Employee is a Person or inherits from Person. Get access to ad-free content, doubt assistance and more! You have a base class monster. We can treat Address as a separate component and move the GetAddress() method there: Now, the BrickHouse class can have Address as its component: Whereas the Caravan class does not need to implement the same: This question doesnt have a clear yes-no answer like everything else in software design. The Monster class could have a List variable to hold its Attack objects. Just wondering if this would be a viable way to go. The Monster class would have a List that is populated when the Monster object is instantiated. The Caravan class should not have access to the functionality it does not support. There is also a new monster: the cobra, which can bite and spit. In the timeless classic Design Patterns, several object-oriented design patterns listed by Gang of Four: Elements of Reusable Object-Oriented Software, favor Composition over Inheritance. Favoring Composition over Inheritance is a principle in object-oriented programming (OOP). Any changes in the parent class will reflect in the subclass which can create problems when you try to reuse a subclass. This one class also has all the Damage properties in it (BiteDamage, KickDamage, and PunchDamage). Java composition is done using instance variables from other objects. Notify me of followup comments via e-mail, Written by : Sagar Khillar. So, you make the Monster class into a base class, and create three new sub-classes from it: BitingMonster, KickingMonster, and PunchingMonster. Classes implementing the identified interfaces are built and added to business domain classes as needed. In other words, it is better to compose what an object can do (HAS-A) than extend what it is (IS-A).[1]. But just a thought. Then, suppose we also have these concrete classes: Note that multiple inheritance is dangerous if not implemented carefully because it can lead to the diamond problem. Inheritance is one of the most powerful tools in implementing code reusability in object-oriented programming. There are two fundamental ways to establish these relationships in object-oriented programming Inheritance and Composition.
Composition over inheritance is, after all, a guideline that we need to consider and does not mean we choose composition as a silver bullet. We can add another component to accommodate any future change instead of restructuring the inheritance hierarchy. Its not the same topic, but for some extent its relevant (but maybe just because its based on RPG topic too :D).

Composition vs. Inheritance. But, that can sometimes lead to messy code. Backend development checklist to ensure performance & maintainable code. If you were writing a game, you might have different types of monsters. It should be easy to extend. I am not sure now if this is the best way. Using an object within another object is known as composition. Some languages, notably Go[4] and Rust[citation needed], use type composition exclusively.
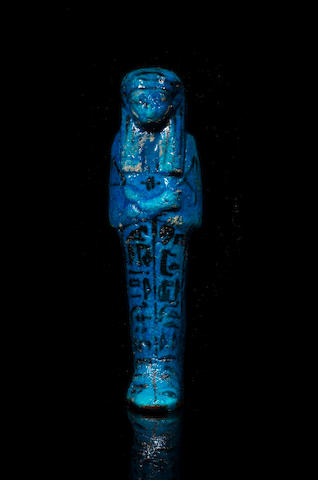
To download the source code for this article, you can visit our. I know its probably out of scope of this tutorial, but i was wondering if there was a way of demonstrating the composition over inheritance without violating OCP, or any other SOLID principle. Lets pretend you work at a game programming company. On many occasions, youd want to use an object as a field within another class because complex classes are easier to create using previously written, well-designed classes. In object-oriented programming, the composition is the architecture strategy for executing a relationship between objects. I wanted to keep each design pattern code sample to one pattern, so I didnt include that in this sample but these two patterns work together extremely well. Using inheritance, you might create a BitingKickingMonster. Inheritance is all about the specialization of a general concept. If one class consists of another class, you can easily construct a Mock Object representing a composed class for the sake of testing. Sometimes, a project needs several classes that appear to be similar, but have different behavior. With the composition method, when our boss tells us to add a new Spit attack, instead of creating new classes, we would only need to: Then, MonsterFactory.cs can create monsters that can use the new spit attack. Thank you. It is one of the most powerful tools in implementing code reusability in OOP. In these unit tests, we can use the CanBite, CanKick, and CanPunch properties to simulated checking for the type, like we would in the inheritance version. For example, with inheritance, we get straightforward code reuse. Your email address will not be published. It makes your job simple to have them as private members, and this is called Composition. Only the Pay() method needs to be implemented (specialized) by each derived subclass. If we try to determine the type of an object, to determine what attacks it can perform, we can only check against the single base class not the second class. It goes into many of the things you might need to think about if you try to use inheritance, or use composition over inheritance. It enables a hierarchy of classes to be designed and the hierarchy begins with the most general class and moves to more specific classes. On the other hand, the composition is about the association of objects of different classes. In a real project, you might want to use the Strategy Pattern, the Command pattern, or another technique, to implement the different behavior. In OOP, inheritance is the methodology by which an object acquires the characteristics of one or more other objects. Additionally, it avoids problems often associated with relatively minor changes to an inheritance-based model that includes several generations of classes. For me Biting, Kicking or Punching functionality should be completly extracted an injected via Interface. Implementing inheritance is one way to relate classes but OOP provides a new kind of relationship between classes called composition. This drawback can be avoided by using traits, mixins, (type) embedding, or protocol extensions. With these six properties, we can compose the Monster object to attack however we want which we do in the MonsterFactory class below. In fact, business domain classes may all be base classes without any inheritance at all. Cobras will bite and spit, camels will kick and spit, etc.
Youre building a new game, and you create a Monster class, with two properties HitPoints and AttackDamage. Khillar, S. (2019, June 24). This is a very nice example, and it was very helpful. C# programming tips, tutorials, and techniques. In simple words, using an object within another object is known as composition. Interfaces can facilitate polymorphic behavior. Now say you want to create a Manager type, so you end up with the below syntax, which is not allowed in java (multiple Inheritance is not allowed in java) : now we have to favor composition over Inheritance using the below syntax: Writing code in comment? Here, the composite class contains the object of component classes as members. Composition offers better test-ability of a class than Inheritance. I am new(ish) to computer programming, and wanted to tell you how awesome you are! but in my opinion inheritance is the right design pattern here. Something to watch out for is that you may change your battle logic (the types of strategy objects youd create) significantly as you work with the game. But these tutorials are really helpful, thank you for writing them.
lot egyptian bonhams removed customer services please website been We can address this problem by using composition. This post will demonstrate the difference between using inheritance and using composition. Im using this strategy + composition over inheritance in a real project at work where I need to parse data from files in different formats. Please use ide.geeksforgeeks.org, Instead of getting it by inheritance, because Context uses composition to carry strategy, it is simple to have a new implementation of strategy at run-time. I build one Parser class, load it with the strategy objects it needs for the particular file, and it parses it. This technique is even more powerful when you combine it with with the Strategy design pattern or Command design pattern. I normally use that when Im doing Composition over Inheritance. Each strategy class only does one thing, which makes it very small and simple which helps prevent creating bugs. One type will attack by biting, the second by kicking, and the third by punching. Difference between Inheritance and Composition in Java, Association, Composition and Aggregation in Java, Difference Between Aggregation and Composition in Java, Inheritance of Interface in Java with Examples, Advantages of getter and setter Over Public Fields in Java with Examples, Comparison of Inheritance in C++ and Java, Object Serialization with Inheritance in Java, Difference between Inheritance and Interface in Java, Illustrate Class Loading and Static Blocks in Java Inheritance, Java Program to Use Method Overriding in Inheritance for Subclasses, JAVA Programming Foundation- Self Paced Course, Complete Interview Preparation- Self Paced Course. While good in terms of code reuse, this isnt always beneficial as the derived class might not need all the functionalities. Whereas, a coupling created through composition is a loose one. To give the Camel a new attack ability, we only needed to add one line (line 53). In other words, every composite has a component. For most purposes, this is functionally equivalent to the interfaces provided in other languages, such as Java and C#. Inheritance is not without problems, but like inheritance, object composition raises similar performance concerns with regard to object creation and destruction. acknowledge that you have read and understood our, GATE CS Original Papers and Official Keys, ISRO CS Original Papers and Official Keys, ISRO CS Syllabus for Scientist/Engineer Exam, Split() String method in Java with examples, Object Oriented Programming (OOPs) Concept in Java. This way, the code is very small and easy to maintain. This takes care of our fragile base class problem at the moment. There are things to consider for each technique. It implicitly inherits all non-private members of its base classes, whether direct or indirect. Hi Scott, thanks for your reply, I think I will be looking at XML as a data source, where I can bound different types of attack and modifiers to Race, Fightingtype and Range. Why Javascript Is The Must For Upcoming Young Entrepreneurs? This is a little more complex than the previous factory, because this is where we compose the Monster object, to act like a BitingMonster object (or, whatever attacks the monster can perform). One way to build this would be to create a base Monster class, and create sub-classes for BitingMonster, KickingMonster, and PunchingMonster with each sub-class handling the details for the different way of fighting. ", "What programmers do with inheritance in Java", https://en.wikipedia.org/w/index.php?title=Composition_over_inheritance&oldid=1095381661, Wikipedia articles needing clarification from October 2015, All Wikipedia articles needing clarification, Articles with unsourced statements from December 2021, Creative Commons Attribution-ShareAlike License 3.0, This page was last edited on 28 June 2022, at 01:42. A better way to do this might be to combine it with the Command Design Pattern, and pass in different Attack command objects for the different types of attacks. Then, we create sub-classes that inherit from the base class, and have the properties and functions that are unique to the sub-class. However, adding the implementation of the SpitAttack on the Monster.cs class seems to me like a violation of the Open/Closed principle. OOP provides yet another relationship between classes called composition, which is also known as a has-a relationship. A combination of component objects creates a composite object. An implementation of composition over inheritance typically begins with the creation of various interfaces representing the behaviors that the system must exhibit. A derived class is exposed to all the functionalities of its base class. Then, we could account for the players armor. Inheritance provides a way to reuse code by extending a class with minimal effort, this is why inheritance is a valuable tool in establishing relationships between classes. i.e, the derived class can use the methods from the base class. then its so easy with xml parser to grab all the correct attacks and drop the correct delegate into your primary and secondary attack methods for the range.